Introduction
To practice Kotlin
- Open Android studio
- Choose a new Empty Activity > Next > Name the project > Choose Kotlin Language > Finish
- Click on tools > Kotlin > Kotlin REPL
- The REPL will appear in the window's bottom
- Press Ctrl + Enter to execute any code
Main Function in Kotlin
// Calling a print line function from main function where it takes an array of strings as its argument
fun main (args: Array<String>){
println("What is your name?")
}
What is your name?
Main function's arguments in Kotlin
// Calling a print line function from main function with arguments and using a string template
// We get the first element of args which is "World"
// We use $ and {} for an expression
fun main (args: Array<String>){
println("Hello, ${args[0]}!")
}
Hello, World
But what is a string template?
//A variable num with value 20
fun main (args: Array<String>){
val num = 20
println("number = $num")
}
//The output
number = 20
//A variable name with value Leila
fun main (args: Array<String>){
val name = "Leila"
println("My name is ${name.length} letters")
}
//The output
My name is 5 letters
In Kotlin, everything has a value
//The value of isPass will be true or false depending on the grade, and in one line
fun main (args: Array<String>){
val grade = 85
val isPass = if (grade > 50 ) true else false
println(isPass)
}
//It can be shorter as well as follows
fun main (args: Array<String>){
val grade = 85
val isPass = grade > 50
println(isPass)
}
//The output will be:
true
fun main (args: Array<String>){
val grade = 85
val report = "you ${if(grade>50) "passed" else "failed"}!"
println(report)
}
//The output will be
you passed!
Random Java library
//Click Alt + Enter to import the java library
import java.util.*
// For candyType function, we have a list of four types of candy & we return a random type using Random. 4 is the upper bound. so it will return any number between 0 & 3.
//For the primary function, we have a variable candy which value is the
function candyType, which will be any string of the four in the available
list.
import java.util.*
fun main (args: Array<String>){
val candy = candyType()
println(candy)
}
fun candyType(): String {
val candy = listOf("Toffee", "caramels", "gummies", "chocolate")return candy[Random().nextInt(4)]
}
Remainder in Kotlin
fun main (args: Array<String>){
val number = 89
val secondNumber = 3
val remainder = number.rem(secondNumber)
val remainderOperator = number % secondNumber
println(remainder)
println(remainderOperator)
}
//The output
2
2
Quiz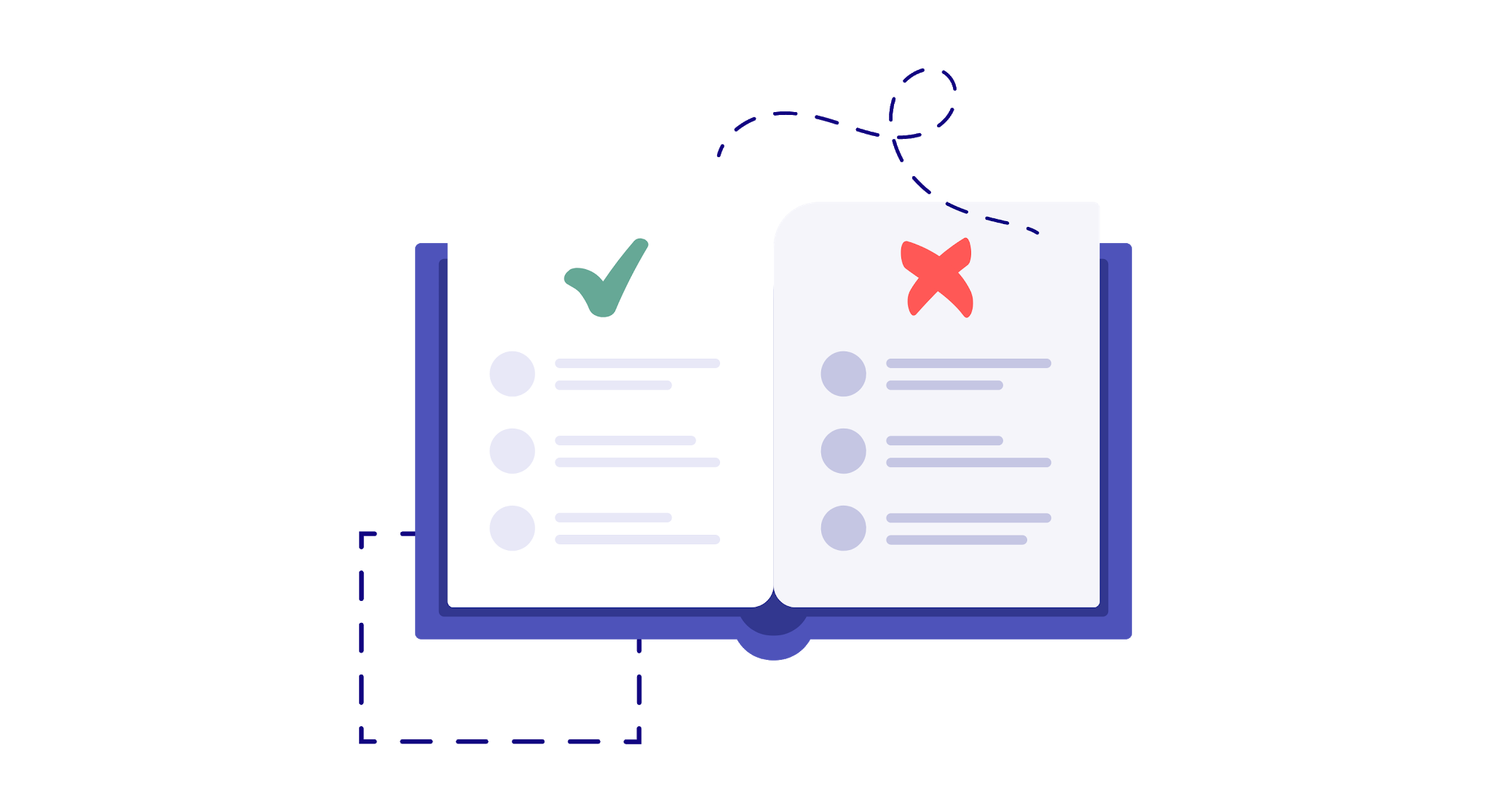
1. What is value of the variable candyChoosen if Random().nextInt(5) is 3?
val candy = listOf("Toffee", "caramels", "gummies", "chocolate", "marshmallow")
var candyChoosen = candy[Random().nextInt(5)]
2. What is the data type of the variable "cold"?
var temp = 45
var cold = temp < 20
3. How do you get a random value between 0 & 5?
4. (True or False)The following two variables will have the same value:
val firstNumber = 56.rem(5)
val secondNumber = 56%5
Thank you for reading my post. You can subscribe to my blog or follow me on Twitter to be the first person to read my new posts.
0 Comments