Introduction
To practice Kotlin
- Open Android studio
- Choose a new Empty Activity > Next > Name the project > Choose Kotlin Language > Finish
- Click on tools > Kotlin > Kotlin REPL
- The REPL will appear in the window's bottom
- Press Ctrl + Enter to execute any code
Basic Types in Kotlin
Operators in Kotlin
//Addition
2 + 2
res0: kotlin.Int = 4
//Subtraction
99 - 12
res1: kotlin.Int = 87
//Division
20 / 5
res2: kotlin.Int = 4
3 / 6
//Integer divided by Integer returns Integer
res3: kotlin.Int = 0
3.0 / 6.0
// Float divided by float returns float
res4: kotlin.Double = 0.5
//Multiplication
6 * 30
res5: kotlin.Int = 180
//Multiplication
3.times(6)
res0: kotlin.Int = 18
//Division
18.div(2)
res1: kotlin.Int = 9
//Addition
9.plus(2)
res2: kotlin.Int = 11
//Subtraction
15.minus(2)
res3: kotlin.Int = 13 reres: kotlin.Int = 9s1: kotlin.Int = 9
Variables in Kotlin
//A variable of type string called firstName of value Leila
val firstName: String = "Leila"
//A variable of type integer called age of value 20
var age: Int = 20
//The type, Int, is inferred in the next line of code
//Once the type of the variable is determined, you cannot change it later.
var age = 20
//The type is needed in the next line of code, since it is not initialized
var age: Int
Basic Types in Kotlin Cont.
val byteNumber: Byte = 2
val intNumber: Int = byteNumber
error: type mismatch: inferred type is Byte but Int was expected
val byteNumber: Byte = 2
val intNumber: Int = byteNumber.toInt()
val threeMillion = 3_000_000
Nullability in Kotlin
val pizza: Int = null
error: null can not be a value of a non-null type Int
//The correct way
val pizza: Int? = null
//Another correct way, here its type is inferred
val pizza = null
//A list of two elements that are Null
var pizzaTypes: List<String?> = listOf(null, null)
//Another correct way
listOf(null, null)
res0: kotlin.collections.List<kotlin.Nothing?> = [null, null]
//A null list
var pizzaTypes: List<String>? = null
//A null list & null elements
var pizzaTypes: List<String?>? = null
pizzaTypes = listOf(null, null)
// If the value of the list is not null, and the elements are not of a nullable type, then the elements themselves cannot be null.
var pizzaTypes: List<String>? = listOf(null, null)
error: type inference failed. Expected type mismatch: inferred type is List<String?> but List<String>? was expected
var cookies: Int? = 4
cookies= null
cookies!!.plus(3)
kotlin.KotlinNullPointerException
Null Testing with Question Mark Operator
//The following line of code means, If drinks is not null, the output would be a value which in this case 7, as dec will decrement the value of drinks so 8-1= 7, but if it was null, the output would have been 0
var drinks = 8
drinks?.dec() ?: 0
res0: kotlin.Int = 7
Quiz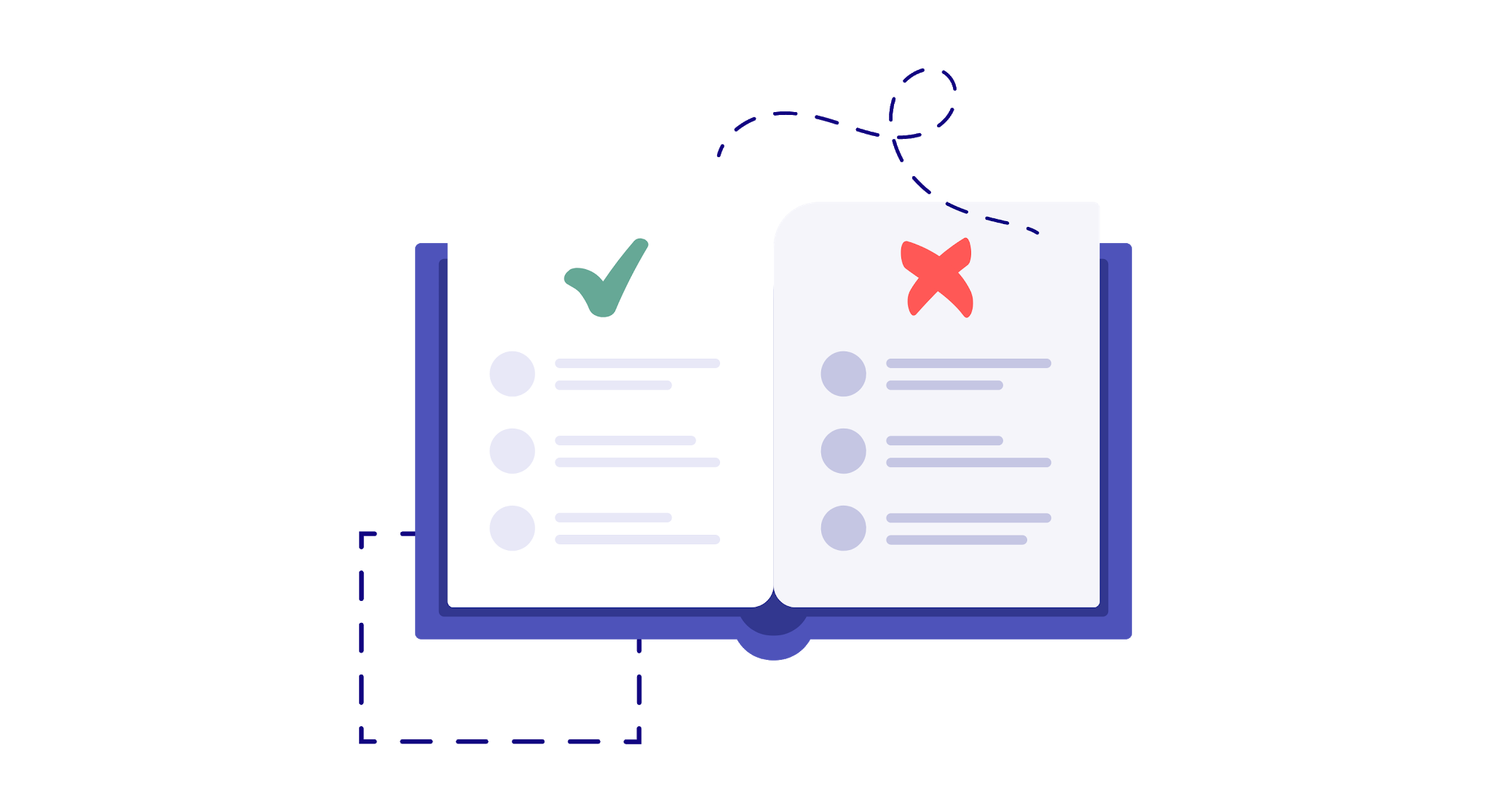
1. What is the output for this line of code: 9 / 2 ?
2. Which Variable keyword you can use that can be reassigned?
3. Which one is the correct syntax?
4. What is the output of this code:
var chips: Int? = null
chips?.dec() ?: 0
Thank you for reading my post. You can subscribe to my blog or follow me on Twitter to be the first person to read my new posts.
0 Comments